Method Overriding in Java?
- If we want to override the implementation of a method in a parent class using child class, we will use method overriding.
- Method overriding is used for runtime polymorphism.
Rules to achieve method overriding:
- Methods in parent and child class have the same name
- Methods in parent and child class have the same type same number of parameters.
- It has an IS-A relationship (inheritance)
public class ParentClass {
public void method1() {
System.out.println("Method1 function");
}
public void multiply(int a, int b) {
System.out.println("Multiply=" + (a * b));
}
}
public class ChildClass extends ParentClass {
public void method1() {
System.out.println("Method1 overrided");
}
public void multiply(int a, int b) {
System.out.println("Overided Multiply function=" + (a * b));
}
public static void main(String[] args) {
ChildClass c = new ChildClass();
c.method1();
c.multiply(10, 20);
}
}
Output:
 |
Method Overriding in java |
Can we override final method ?
- No. final methods can't be overriden. If we try to override compiler throws exception.
 |
- can we override final method
|
Can we override static method ?
No. we can't override static methods.
Calling of static method depends upon the type of obejct that calls static methods:
- If parent class object calls static method then parent class static method will be invoked.
- If child class object calls static method then child class static method will be invoked.
Method overriding is based on dynamic binding at runtime whereas static methods are using static binding at compile time.
public class ParentClass {
public static void method2() {
System.out.println("Parent class static method");
}
}
public class ChildClass extends ParentClass {
public static void method2() {
System.out.println("child class static method");
}
public static void main(String[] args) {
ParentClass p=new ChildClass();
p.method2();//ParentClass.method2();
ChildClass c = new ChildClass();
c.method2();//ChildClass.method2();
}
}
Output:
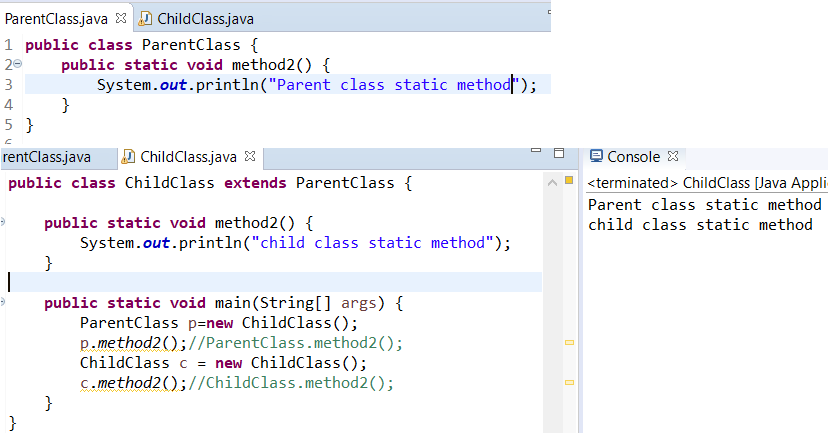 |
can we override static method |
Please comment below to feedback or ask questions.
No comments:
Post a Comment
Please comment below to feedback or ask questions.